Android Course Overview

The goal of Android App Development Training course is to provide developers easy and complete understanding of the Android App Development with our Class Room Training. The Android Training course provides a series of sessions & Lab Assignments which introduce and explain Android features that are used to code, debug and deploy Mobile Applications
Pre-requisites of the Course
1.Development in Java Programming Language
2.Understanding of application development frameworks, environments, tools and processes
Android Training Objectives
1.Upon completion of this course, attendees will be able to
2.Understand Android platform architecture
3.Design, develop, debug, and deploy Android applications
4.Use Android SDKβs Emulator to test and debug applications
5.Construct user interfaces with built-in views and layouts
6.Define custom view and layout
7.Develop SQLite Data base
8.Secure Android applications
9.Write multimedia Android applications
10.Write location-based applications
11.Interact with Servers using Web Services
Course Duration
45 Working days, daily 1.30 hours
Android Training Course Overview
Introduction to Android
1.Overview of Android
2.Java Editions and comparison with Android
3.Android Apps β Design, Vendor, Behavioral Classification
Android Architecture Overview
1.Android Architecture
2.Application Frameworks
3.Android Libraries, Run time, Dalvik Virtual Machine
Setup of Android Development Environment
1.System Requirements
2.Java, Eclipse and Android SDK Installation
3.Android SDK andTools
4.Android Virtual Devices & Device Definitions
Your Android Application
1.Android Application Design
2.Using PhotoShop for Graphic Designing
3.Android Application Wireframes (screens)
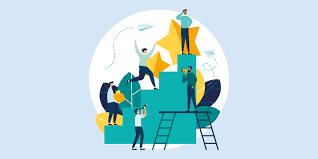
Your First Android Application
1.Creating Android Application
2.Creating Configurations
3.Testing the app: AVD, Active Device
4.Android Project Structure and Manifest file
Publishing to the Play Store
1.Release process and Release build of Android Application
2.Signing the .apk file
3.Preparing the Store Listing page
4.Content Rating
5.Distributing the Application
6.Merchant Registration for Paid Applications
Activities
1.About XML β approach to design layouts
2.Views and Layouts
3.View properties
4.Linear Layout vs. Relative Layout vs. Frame Layout vs. Absolute Layout
5.Localization of UI
6.Best practices for targeting various form factors: phone, tablet, TV
7.Best practices when working designing Android UI
Android Testing
1.Creating a Test Project for Android project
2.Working with Test Packages
3.Writing test cases
Fragments
1.Designing fragments
2.Fragments life cycle
3.Fragment management and integration

User Interfaces
1.Creating the Activity
2.XML versus Java UI
3.Selection Widgets, Using fonts
4.Common UI components
5.Handling UI events: a bit about listeners
Advanced UI
1.Adapters
2.Complex UI components
3.Menus and Dialogs
4.Tabbed Activities
5.Navigation Drawer
6.Animations
7.Create activity layouts programmatically
8.Testing and optimizing UI
Android Material Design
1.What is material ?
2.Material properties and Styling / Animations
3.Material Patterns
4.Resources
5.Overview of Android Resources
6.Creating Resources
7.Using Resources
8.Drawable Resources
9.Animation Resources
Broadcast Receivers
1.Broadcast receiver usage patterns: when and why to use them
2.Implementing a broadcast receiver
3.Registering a broadcast receiver via the manifest file and Programmatically
Background Services
Overview of Android services
Service lifecycle
Declaring a service
Registering a service
Starting and stopping a service
Threads and other concurrency considerations with services
Bound versus unbound services
Remote versus local services
Intents
Working with Intents
Explicit and implicit intents
Using Intents as messaging objects
Intents to start components expecting results
Storing and Retrieving Data
Storage Model selection criteria
Shared preferences
Internal Storage β Files
External Storage β SD Card
Testing the created files, tools
SQLite Database
Introducing SQLite
SQLiteOpenHelper and creating a database
Opening and closing a database
Working with cursors
Inserts, updates, and deletes
Native Content Providers
Content provider types
Searching for content
Adding, changing, and removing content
Native Android Content Providers
Accessing Contact Book, Calendar
Custom Content Providers
Custom Content Provider classes
Publishing content providers
Web Services
Understanding Web Services
Web Services Architecture
Building Server side components
Publishing web services
REST based web services
Accessing Web Services
Integrating Web Services with mobile client
Overview of networking
Checking the network status and web service status
Working with HTTP to access the web services
Parsing, Parsers
Document Object Model ( DOM )
Simple API for XML ( SAX )
JavaScript Object Notation ( JSON )
Parsing XML and JSON
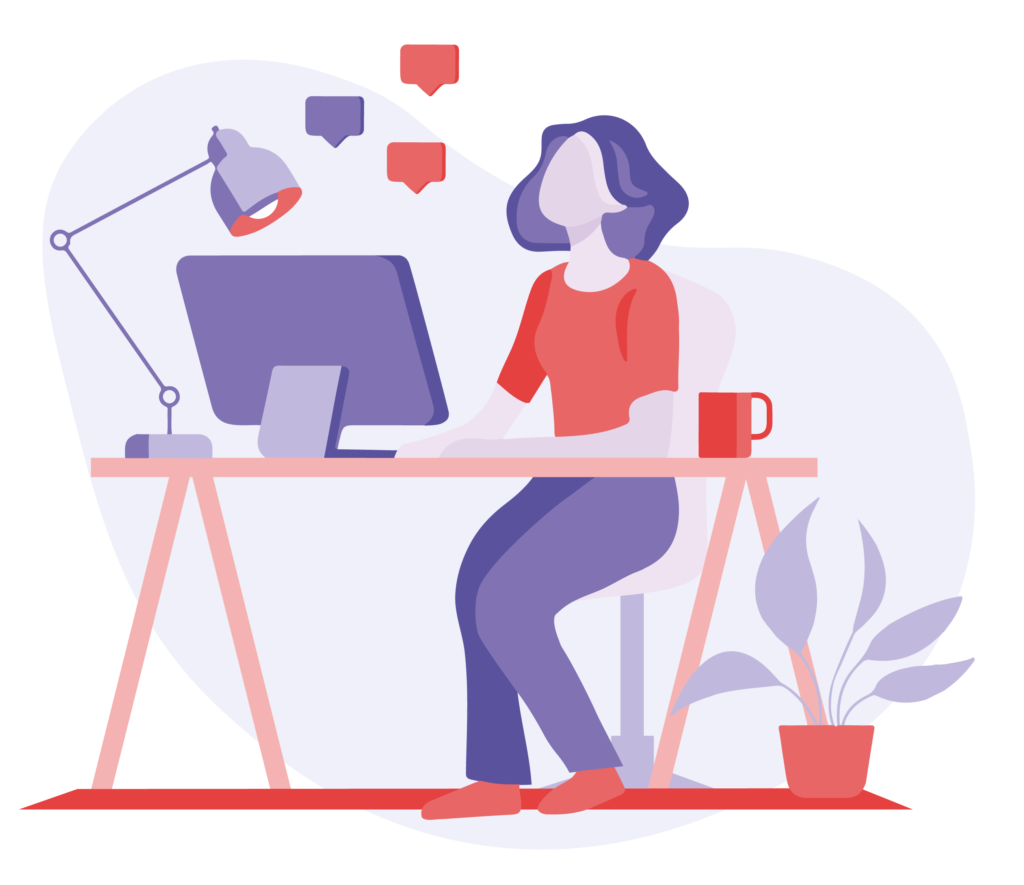
Location Based Services
Using Location Manager, Location Provider
GPS and Network based tracking
Testing the application using KML files
Simulation of the locations on the active device
Location Listeners and Proximity Alerts
Integrating Google Maps
API Version 2 of Google Maps
User Interface β MapFragments
API key generation
Registrations in the manifest file
Google Map, Camera Positions
Adding Markers, Circles, Polylines
Google Maps Directions API
Telephony
Telephony background
Accessing telephony information
Monitoring data activity and connectivity
Working with messaging SMS
Multimedia in Android
Playing Audio & Video
Recording Audio & Video
Customizing Camera & Capturing Photos
Voice Recognition
Text To Speech
Bluetooth
Controlling local Bluetooth device
Discovering and bonding with Bluetooth devices
Managing Bluetooth connections
Communicating with Bluetooth
Social Networking Integrations
Facebook Integration
Debugging and Testing Android Apps
Logcat
Debugger
Traceview
HierarchyViewer
Monkey Runner
UIAutomator
Mobile apps have changed our way of life, from the way we run our businesses, educate, recreate and find entertainment! This has made mobile app development one of the most fascinating and coolest jobs around. Android is indeed, the indisputable leader of global mobile market share. This market leadership implies high job security for Android developers.
As Android apps continue to connect people across the globe and enable users to engage in more innovative and interesting ways, a career in Android development has become ever more enriching, fulfilling and in demand to the global economy. To become a successful Android developer, you need to develop several skills and apply them at the right place and time to provide the best experience to your users.
Here are the 10 essential skills you need to succeed as an Android developer.
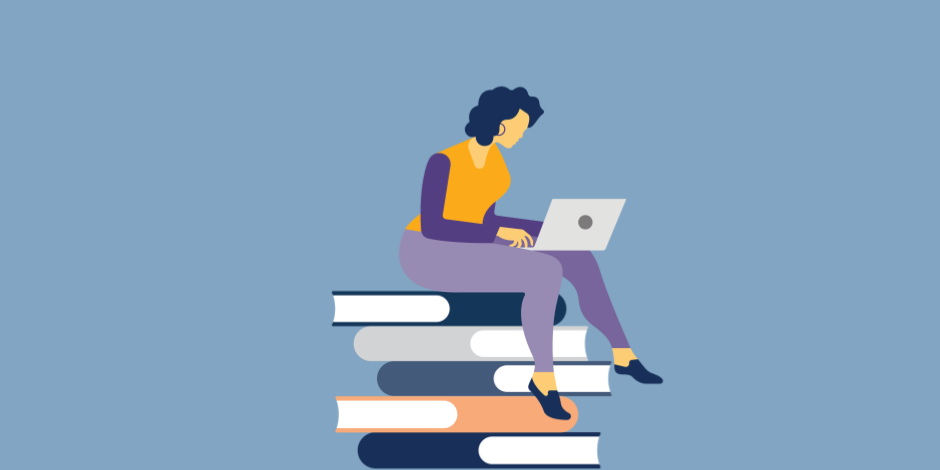
- Android foundations
The most basic building block of Android development is a programming language. The most preferred languages to create Android apps are Java and Kotlin. You can use either Java or Kotlinβor even both at the same timeβto develop apps. You need to be familiar with core concepts of one or both of these two programming languages, including basics and syntaxes, collection framework, concurrency and multithreading, generics and functional programming.
To design layouts (user interface) in Android, we use XML. Much like HTML, XML is also a markup language and stands for eXtensible Markup Language. After creation of these XML Layouts, they are linked to Kotlin/Java files where business logic is written.
To design seamless XML layouts and write business logic in an integrated environment, there exists a handy tool known as Android Studio. Android Studio uses Gradle, an advance build toolkit, to automate and manage your build process. To integrate Gradle in Android studio there exists a plugin known as Android plugin for Gradle. Before you get started, you need to understand what Gradle is and how to use it specifically for managing external dependencies.
Gradle and Android plugin run independently of Android Studio. This means you can build your Android apps from within Android Studio, the command line on your machine, or on machines where Android Studio is not installed (such as continuous integration servers). If you are not using Android Studio, you can learn how to build and run your app from the command line.
- Android interactivity
User interaction with Android apps should be considered with utmost priority. Responding to events by using callbacks, implementing appropriate gestures such as drag and drop, providing right keyboard for user input, and using pan and zoom in the right place plays a crucial role in providing the seamless user experience. These are really small but very useful in improving user interaction. Above all, these user interactivity takes place in an Android component known as Activity.
An Activity is a single, focused thing that the user can do. Almost all Activities interact with the user, so the Activity class takes care of creating a window for you in which you can place your user interface.
The Android device comes with various screen configurations. Screen orientation is one of them. It can be either portrait or landscape. Each Activity can exist in either portrait or landscape mode. When the screen is rotated the current activity is destroyed and recreated in a new orientation. The stability of an Activity should always be maintained when screen orientation changes.
- Android UI
An application is incomplete if it lacks modern UI. An application may contain useful functionalities but if it fails to adapt to modern UI guidelines then it is ultimately doomed to fail.
Using RecyclerView to implement lists and grids, using ConstraintLayout for designing complex layouts, applying animations to enhance user experience, using appropriate menus, following Material design guidelines and using vector drawables instead of raster graphics are few of the basic rules that every developer must follow in their apps.
While designing layouts one must not forget to consider the multiple screen type devices. The same app should look different for different devices. For example, the screen on a tablet should show dual-pane layouts to effectively utilize a widescreen. While on a smartphone it should use single pane. This behavior can be achieved by using Fragments. Additionally, in several cases, you might need to customize Views that suit your need. This can be achieved by designing custom components for your Views.
- Implementing navigation
Navigation refers to the interactions that allow users to navigate across, into and back out from the different pieces of content within your app. There are various UI elements that allow you to implement effective UI navigation.
The most basic one is the app bar which is popularly known as Toolbar. On your Toolbar, you can add a popup menu, menu icons and add navigation drawer icon. This navigation drawer icon helps you to open and close the navigation drawer which is another element to implement effective navigation. The navigation drawer allows you to add additional menu items which can help you to swap fragments in your current Activity or perform some other relevant actions.
Another navigation element is a BottomNavigationView which allows you to switch between different Fragments in the same Activity by using tabs at the bottom of the screen. If you need to use tabs attached to the Toolbar and swipe between Fragments (different pages), then ViewPager should be your choice.
User intention is the most important aspect of navigation. The user might want to launch new Activity, move back to the previous Activity, or even share data to some other app. These intentions can be achieved by using Intents in Android. If you know your target Activity use Explicit Intent otherwise use Implicit Intents. You are always allowed to configure your intents.
Above all, Android Jetpack’s Navigation component helps you implement navigation, from simple button clicks to more complex patterns, such as app bars and the navigation drawer. You might want to take a look at this component before you start implementing navigation in Android.

- Android testing
Users interact with your app on a variety of levels, from pressing a Submit button to downloading information onto their device. Accordingly, you should test a variety of use cases and interactions as you iteratively develop your app. Most commonly used testing libraries are Expresso, Junit, Mockito, Robolectric and UI Automator. - Working with data
Data is an important part of your application and must not be ignored. You may need to fetch data from the server, query data from the local database, save user preferences and do file handling.
To use local database prefer using Room database which is the part of Android Jetpack’s architecture component. You might want to share your database outside your app securely using ContentProvider.
To save a small amount of data or save user preferences in the form of key-value pair you can use Preferences API, which is based on SharedPreferences API.If you want to bind your UI components in your layouts to a data source in your app using a declarative format use Data Binding Library.
To handle files in your app you can take advantage of massive file system APIs provided to you.
Above all, to access data over network (i.e. while consuming REST APIs) use Retrofit 2 library which is a blessing to all Android developers by Jake Wharton.
- Notifications
Notifications are one of the best ways to increase user engagement. It appears outside of your app’s UI to provide the user with reminders, communication from other people or other timely information from your app. Users can tap the notification to open your app or take an action directly from the notification. You can create a notification, customize its layout, add action to it and even group multiple Notifications using Notification channels. - Firebase on Android
If you hate being a backend developer, Firebase is the way for you. Firebase is a mobile platform that helps you quickly develop high-quality apps, grow your user base and earn more money. Firebase provides several utility features to make the life of an Android developer easy.
To make your app visible in Google search you can use Firebase App Indexing API. You can use cloud storage and cloud firestore to store data in your server. If you need to automatically run backend code in response to events triggered by Firebase features and HTTPS requests, use Cloud Functions. Next is the Firebase Cloud Messaging (FCM) that lets you push notifications to your clients. Using FCM, you can notify a client app that new email or other data is available to sync. Use Firebase Crashlytics, which is a lightweight, real-time crash reporter that helps you prioritize and fix stability issues. If you have ever used deep links in your app, try Dynamic links. With Dynamic Links, your users get the best available experience for the platform they open your link on.
You might want to take a look at other Firebase features such as Firebase Invites, Firebase Performance Monitoring and Firebase Remote Config.
- Android security
Android has built-in security features that significantly reduce the frequency and impact of application security issues. You can protect the user’s privacy by using permissions. Share data securely by using signature-based permissions. Handle data security by using internal storage, external storage and ContentProviders very cautiously. By default, never export your Android component such as ContentProvider, Service or BroadcastReceiver unless you need to.
Android has limited the capability of WebView to minimum functionality to avoid web security issues such as cross-site scripting (JavaScript injection). But still you need to be cautious and minimize the frequency of asking your user’s credential. Instead, use an authorization token and refresh it at regular intervals.
- Git: Version control system
Git is free and open-source software used for version control. Git lets you experiment with new application features and coding techniques with confidence. You can work on new crazy ideas for your project while your previous working copy stays safe and secure. The moment you may realize you are evolving your project in the wrong direction, you can restore your previous version, hassle-free, and course correct.
As the world moves ever more into the mobile landscape, Android app developers can be sure to find an audience for their creations, or bring great value to companies in need of mobile app development skills.

Why choose us Coding Trainings
1. Having More Than 15 Years Of Experience In Medical Coding Training
2. 13+ Years Of Experience In Real-Time Industry
3. 100% Lab Facility For Every Student By Experienced Lab Coordinators
4. Training On Live Projects And Assignments
5. 100% Placement Assistance
6. Lifetime Access To Latest Content
7. 24 X 7 Trainerβs Support On Online Forum
8. Giving Credits For Real Time Internship
9. Get Full Access To Paid SEO Tools
10. Become A Certified Professional
How much Does Medical Coding Training Fees Hyderabad
Fees for Medical coding 6,000+ only if ur Looking for All training 12,000+ Classroom training and internship training with live projects
S Nandini reddy
β
β
β
β
β
1 days ago
Best institute from recent times to learn CPC with live projects Nice
Reply Like
shiva bkb
β
β
β
β
β
8 days ago
Well experienced faculty for medical coding highly recommended for training
Reply Like
javeed ahmedξ€³ξ€³ξ€³ξ€³ξ€³